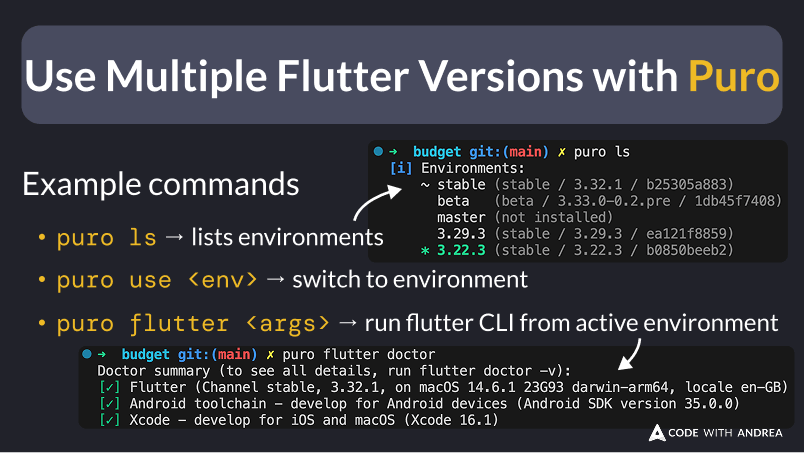
Use Multiple Flutter Versions with Puro
1 min read
Puro is a powerful tool that lets you install multiple versions of Flutter and easily switch between them in your projects.
Puro is a powerful tool that lets you install multiple versions of Flutter and easily switch between them in your projects.
Dart 3.8 introduces a new formatting option to preserve trailing commas, overriding the new formatting style introduced in Dart 3.7.
Dart 3.8 introduced an updated formatter with various configuration options. Learn what’s new and how to prepare your codebase.
Null-aware elements allow you to add nullable elements to a collection with a single character (?).
To enable this, switch to Flutter 3.32 and run your app with --web-experimental-hot-reload
If your app uses GoRouter with shell routes, tracking screen views reliably requires some workarounds. Here's how to make it work.
Material 3 supports 5 types of buttons: elevated, filled, filled tonal, outlined, and text. Here's how to use them in Flutter.
By overriding ThemeData.platform inside MaterialApp, you can quickly test all your adaptive widgets and any conditional code that checks the platform.
A complete guide to adding analytics to Flutter apps using Firebase. Track custom events with a maintainable architecture.
A simple alert dialog that supports adaptive mode, default and cancel actions, destructive style, and dismissible mode.
New Kotlin DSL in Flutter 3.29? Learn how to update your Gradle files, configure code signing, flavors, and more, in minutes.
Use this script to update the Gradle, Java, NDK version and other settings in your Android project.
A battle tested solution for loading Firebase Remote Config in Flutter, avoiding common pitfalls.
debugRepaintRainbowEnabled helps you discover widgets/areas that unexpectedly repaint in your app. Here's how to use it.
debugRepaintRainbowEnabled helps you discover widgets/areas that unexpectedly repaint in your app. Here's how to use it.
When working with forms in Flutter, numeric inputs need special attention. To improve the user experience, set the appropriate keyboardType and inputFormatters.
The TextSpan class lets you set a custom mouse cursor style, along with a tap gesture recognizer for opening your URL links on Flutter web.
Learn how to set up Firebase for multiple flavors in your Flutter app using the FlutterFire CLI. This guide covers iOS, Android, and web configurations.
A/B tests help you make data-driven decisions and increase conversions in your app. Here's how they work.
Static release toggles let you release unfinished code without activating it in production. Here's how to use --dart-define to manage them.
When reading variables from .env files, you can use int.fromEnvironment and bool.fromEnvironment to read integers and booleans.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Here's a handy command to override the Android status bar UI before taking screenshots for your Play Store listings.
Here's a handy command to override the iOS status bar UI before taking screenshots.
Apps created with Flutter 3.29 use the new Gradle Kotlin DSL on Android. Some CLI tools don't support the new syntax, yet.
Pub.dev has a new chart that lets you see package download counts by version (major, minor, patch). Here's where to find it.
A real-world case study about ephemeral vs application state, navigation, scroll controllers, and how refactoring can help you ship new features smoothly.
Pub.dev has a new chart that lets you see package download counts by version (major, minor, patch). Here's where to find it.
By registering a ValueNotifier listener, you can perform side effects such as updating controllers, showing dialogs, and performing navigation.
Dart 3.7 introduces a new formatter that automatically adds or removes trailing commas, based on the max line length.
Since Dart 3.7, the _ character is a wildcard variable and you can use it more than once in your code, without causing name collisions.
The CallbackShortcuts widget makes it easy to add keyboard shortcuts to your Flutter app. Here's how to use it.
Here's how to create a responsive split-view widget that works on mobile, desktop and web.
If you're using GitHub Actions for your CI/CD needs, you can setup a self-hosted runner to cut your build times and save money.
If you add a debugFillProperties() method to your custom widget classes, all your custom widget properties will show in the DevTools.
A reference guide for releasing your Flutter app on the Play Store, including app content, store listing, Android project settings, and code signing.
With VSCode, you can easily move your Dart classes and functions to a different file (this is very useful when refactoring).
Here's how to overlay multiple widgets inside a Stack and constrain their size and position with Positioned and FractionallySizedBox.
If you need to select between a small list of values but have limited vertical space, you can use a ListWheelScrollView.
To support the latest wide gamut color spaces, Flutter 3.27 has deprecated some properties and methods in the Color class.
If you want to render fixed width (monospaced) digits, set FontFeature.tabularFigures() inside your TextStyle.
Since Dart 3.6 (Flutter 3.27), you can use _ as a digits separator. This works with integers and floats, as well as custom formats (hex, scientific).
Since Flutter 3.27, you can pass a spacing argument to your Row and Column widgets (rather than using SizedBox).
You can use the Banner widget to place a small diagonal banner over a child widget. For more custom styling, build your own using a custom painter.
You can use Cursor edit mode to sweep through your code and make changes. Works best with imperative code.
A few suggestions for solving the "version solving failed" error when updating your Flutter dependencies.
A step-by-step guide on how to publish your Flutter app, including metadata, compliance, privacy manifests, Xcode settings, and building your IPA file.
Starting November 12, 2024, apps that don’t include a Privacy Manifest can’t be submitted for review in App Store Connect.
Some useful Riverpod techniques you can use to initialize async dependencies, show some loading UI, and handle errors during app startup.
If your app does not use Non-Exempt Encryption, set ITSAppUsesNonExemptEncryption to NO in your Info.plist file in Xcode.
Upgrade all your file icons with the Material Icons Theme. File nesting is properly supported, too, and the icons will be correctly left-aligned.
A simple script to build and upload your iOS app to App Store Connect. You can run this locally, no CI/CD needed!
Some guidelines to help you decide which API keys belong on the client, and which belong to the server.
How to enable dark mode and see downloads count for your favourite packages on pub.dev.
With Error.throwWithStackTrace, you can throw custom exceptions while keeping the original stack trace intact.
If your app sales are less than $1M/year, you can apply to the Small Business Program and slash your fees to 15%!
Since Riverpod 2.6.0, all generated providers can be declared with a Ref argument. Here's how to migrate to the new syntax.
If you want to upgrade all dependencies to the latest non-major version, ignoring the pubspec.lock file, use flutter pub upgrade.
An overview of two different strategies for initializing Firebase inside a Flutter app with multiple flavors.
If you have a Flutter project that no longer builds on a specific platform, you can delete the whole folder and generate it again.
If you ever needed a force update prompt that is controlled remotely, you can use the force_update_helper package.
Your Flutter app should show the licenses for packages in use. This is often a legal requirement, as many open-source licenses require attribution.
Prepare your Flutter app for launch with these 6 steps, including flavors and environments, error monitoring, force updates, and in-app reviews.
How to enable dark and tinted icons on iOS 18 using the flutter_launcher_icons package.
If your Flutter app uses multiple flavors, you can use the FlutterFire CLI to generate the config files for each flavor.
Here's how to remotely control the behaviour of your app by fetching some JSON from a GitHub gist.
If you're not too picky about how you write your commit messages, this can be a neat little time saver!
If you're using multiple code generators that depend on each other, you can enforce the code generation order in your build.yaml file.
If you want to return a stream that depends on some asynchronous code, you can use async* and yield*
The in_app_review package makes it easy to ask for reviews. And by using a data-driven approach, you can show the prompt at the right time.
To avoid prompting users too early, track your desired event and only ask for a review after it is triggered N times.
How to download multiple releases from xcodereleases.com, and switch between them with the xcode-select CLI.
By implementing a NavigatorObserver, you can track page views or add navigation breadcrumbs to your error logs.
The Android debug logs can get quite noisy. To work around it, open the DevTools logging page, which will only show the Flutter logs.
Rather than calling Navigator.of(context), you can define all the push/pop methods inside a BuildContext extension and make your navigation code less verbose.
To create a stylish Text, use the ShaderMask widget with a shaderCallback that returns a LinearGradient with begin and end points, along with a list of colors.
The easiest way to add a badge to an IconButton is to use the Badge widget. Use this to show a numeric value or a custom label next to an icon.
Thanks to RawGestureDetector and SerialTapGestureRecognizer, you can implement a custom TripleTapDetector widget.
How to use the Flutter VSCode sidebar to access the DevTools and other useful functionality.
Since Flutter 3.24, a new Rebuild Stats feature is available on the DevTools performance page. Use it to spot widgets that rebuild too often.
Since Flutter 3.24, a new CarouselView widget is available. You can set the children's size with itemExtent and shrinkExtent, and use it with any widgets as children.
As of Flutter 3.24, a new "pub unpack" command is available. You can use it to download a package locally and easily explore its source code.
OverflowBar makes it easy to layout your widgets in a row unless they overflow the available horizontal space, in which case they're arranged as a column.
When you call a method that returns a Future, you have to choose between using await, unawaited, and ignore. Here's an explanation.
The humble log function has many arguments that can be used to customize the appearance of your logs. Here's how to use them.
When tracking analytics events in your code, consider using `unawaited` from `dart:async` (analytics calls should be fire & forget).
--dart-define-from-file supports both .env and json files. Here's how to use each variant.
By doing some string manipulation on the current stack trace, you can extract the current method name (useful for logging).
The hugeicons package was recently released, featuring a collection of over 4,000 stroke Flutter icons.
An overview of different techniques for storing API keys on the client, along with security best practices to prevent them from being stolen.
You can use CancelToken to stop unnecessary data fetches when users navigate away or to implement "cancel" buttons in your Flutter apps.
You can use BoxHeightStyle to change the selection height style of a TextField (useful for multi-language apps).
The widget build method can potentially be called in every frame and should not have any side effects.
If you need to show a semi-transparent image, use the opacity argument with AlwaysStoppedAnimation rather than adding a parent Opacity widget.
Forgetting to dispose controllers in your State class can lead to memory leaks, and the "dispose-fields" rule from DCM can warn you about it.
With this VSCode extension, you can remove all the unused assets, files, and dependencies in your Flutter project and decrease your app bundle size.
If you try to access a web-specific API on native platforms, your app will crash. Here's how to use conditional imports to solve this problem.
To detect the current platform, check for kIsWeb beforehand and use it together with defaultTargetPlatform, which doesn't import dart:io.
With the Universal Platform package, you can perform platform detection with a unified syntax on all platforms, including web, but there's a catch.
A collection of useful aliases to speed up your Flutter app development workflow.
How to use the Flutter CLI to save screenshots from connected iOS and Android emulators
A curated list of popular VSCode shortcuts, extensions & settings to speed-up your Flutter development workflow and code like a pro.
Here's how to use typedef to define type aliases for your function and non-function types in Dart.
By adding type annotations to your collections, the Dart analyzer will warn you if you add values of the wrong type.
Const is for hardcoded, compile-time constants. Final is for read-only variables that are set just once. Var is for variables that are set more than once.
How to use the web app bootstrap process (new in Flutter 3.22) and add a CSS progress indicator before the Flutter app is ready to take over.
Here's how to use the Vector Graphics Compiler to precompile SVGs at build time for better rendering performance.
How to use the JsonCodable macro to augment your classes with fromJson and toJson methods
If you need to return an empty widget, SizedBox.shrink() is more performant than an empty Container.
By default, asserts are only enabled in Debug mode. To enable them in Release mode, run with the --enable-asserts flag.
How to send HTTP requests and view the response directly in Visual Studio Code.
If you use build_runner and your generated Dart files are added to Git, you can hide them by default in your PRs and diffs. Here's how.
Some helper widgets for creating Flutter layouts that grow horizontally up to a given width, then remain fixed at that width.
Ever wanted to filter tests so you only run the ones you need to? This can be easily done using test tags.
An extensive guide covering the code generation mechanism in Dart, useful code-generating packages, and tips for efficient codebase maintenance.
You can run a widget test multiple times with the variant argument. Very useful for golden image tests for different screen sizes.
Create small, reusable widgets that are easier to reason about, and banish the massive build method from existence!
In Flutter you can use DecoratedBox to set a lot of decoration/styling options to your widgets. Here's how.
The faker package lets you generate addresses, names, food, dates, sports... you name it! Here's how to use it.
A useful tip to write more performant code when using SizedBox as a gap between widgets inside a Row or Column layout.
If you have many AsyncNotifier subclasses, using try/catch can be tedious. With AsyncValue.guard you get the same result with less boilerplate.
How to style an ElevatedButton in Flutter, including reusing the same style across all buttons with ThemeData.
How to add a GitHub Actions workflow to automatically run tests when you push your code.
How to easily add fixed spacing inside Flex widgets such as Columns and Rows or scrolling views.
A complete guide to implementing pagination with Riverpod, covering: infinite scrolling, loading and error states, search UI, caching, and debouncing.
Learn how to build and deploy a Dart Shelf App that taps into the OpenAI API without exposing the API key in your Flutter client.
A step-by-step tutorial showing how to deploy your Flutter web apps to GitHub Pages using Makefiles.
Is FlutterFlow the real deal? Or should you ignore it and continue building apps the old-fashioned way? Here's my take as an experienced developer.
A tour of the new Copilot features. Learn how its AI assists with code, tests, and commits, and find out if it's right for your Flutter projects.
A step-by-step tutorial showing how to implement deep links in Flutter using GoRouter, including the native Android and iOS platform setup.
Find out when to use one-time reads and when to switch to realtime updates in Flutter development for optimal app performance and user experience.
An introduction to webhooks for Flutter developers, showing how to create, register, and secure a Stripe webhook using Firebase Cloud Functions.
When building mobile apps, we often need to fetch and mutate data. This article explains how to do it effectively using my reference Riverpod architecture.
How to implement controller classes that can hold business logic, manage widget state, and interact with repositories in the data layer.
Comparing my Riverpod App Architecture with other popular ones such as MVC, MVVM, Bloc, Stacked, Clean Architecture, and Android App Architecture.
Introducing a new Riverpod App Architecture that can be used to build scalable and maintainable apps with a clear structure.
An in-depth explanation of the difference between Go and Push when using GoRouter for declarative routing.
Learn how to parse JSON and define type-safe model classes that can handle validation, nullable/optional values, and complex/nested JSON data (updated to Dart 3).
How to implement bottom and side navigation with stateful nested routes in Flutter using the GoRouter package (example app with source code included).
How to use sealed classes to create a Result type that makes it explicit when a function can return an error, rather than throwing an exception.
Getting a Flutter & Firebase app to work on macOS is a tricky endeavour. Follow this step-by-step guide to get it working.
An overview of the major features introduced in Dart 3, including: records, patterns, switch expressions, sealed classes, and class modifiers.
An in-depth overview of what abstractions to use to write backend-agnostic code that is more testable and maintainable, along with their tradeoffs.
An overview of Firebase as a BaaS platform, including available features, supported platforms, pricing, portability, documentation, and developer experience.
A simple guide showing how to use the FirestoreListView widget to enable pagination when loading large collections of documents from Firestore.
The new Riverpod Lint package adds useful lints and refactoring options that make writing Flutter apps a breeze. Here's how to make the most of it.
A step-by-step guide showing how to migrate StateProvider and StateNotifier to the new Notifier and AsyncNotifier classes (StreamNotifier is covered too).
Thanks to the new Riverpod Generator package, we no longer have to declare providers manually. This guide explains how to use the new @riverpod syntax.
AsyncNotifier does not have a mounted property and this can cause some problems in practice. This article explores some solutions and their tradeoffs.
Since Flutter 3.7, BuildContext has a mounted property that we can check after an asynchronous gap. Here's how to use it.
Let's explore some techniques to separate our business logic and navigation code from the UI, using GoRouter and Riverpod.
An overview of what Dart Fix can do for you, along with some useful VSCode productivity tips and settings for Flutter app development.
I asked ChatGPT to explain some code and write several programs in Dart & Flutter. Here's a full report with my findings, and some tips to make the most of it.
Riverpod is a powerful reactive caching and data-binding framework. Let’s learn how to make the most of it so we can use it effectively.
AsyncNotifier is great. But how do we write unit tests for it? Here are all the details and a template you can follow to test your AsyncNotifier subclasses.
A complete guide to the Riverpod package as a reactive caching and data-binding framework. Fully updated to Riverpod 2.0.
How to access localized strings outside your widgets without a BuildContext, by creating a locale-aware AppLocalizations provider using Riverpod.
Flutter apps built for macOS need a client network entitlement in order to make network requests. Here's how to configure it.
Fpdart aims to bring all the main types found in functional languages to Dart. Here we focus on the Either type and learn how to use it for robust error handling.
An overview of how we can use Riverpod to register listeners and initialize complex objects with dependencies during app startup.
An introduction to singletons in Flutter: what problems they solve, what other ones they introduce, and what are their alternatives.
Service classes are the ideal place to store logic that depends on multiple data sources or repositories. Let's explore them by building a shopping cart feature.
Asking questions and receiving answers is a two-way street. Here's what to do when you need to ask a technical coding question.
DartPad makes it easy to share your Dart & Flutter samples using GitHub gists, and you can even embed them on your website. Here's how.
When a test waits for a stream value that is never emitted, it will timeout after 30 seconds (by default). Here's how to make it fail fast with a custom timeout.
The Flutter testing APIs offer some powerful stream matchers and predicates that we can use to verify state changes over time. Let's see how to use them in practice.
Since Dart 2.17, you can initialize parameters of the super class with a new shorthand syntax. Here's how.
Since Dart 2.17, we can add members and additional methods when declaring an enum. Here's how.
When writing tests for functions that throw, we should not invoke them directly, but rather pass them as arguments using a tear-off.
Ever needed to update a value if a given key already exists, or set it if it doesn't? Here's how to use the Map.update() method to solve this.
How to use the flutter tool generate a test coverage report for your app or package, and improve your testing workflow with two helpful VSCode extensions.
An overview of the feature-first and layer-first approaches when choosing a project structure for medium/large Flutter apps, along with their tradeoffs and common pitfalls.
The AsyncValue class from the Riverpod package offers a much nicer API compared to AsyncSnapshot from the FutureBuilder and StreamBuilder widgets. Here's how to use it.
An introduction to the domain model and its role in defining entities and the business logic for manipulating them in the context of Flutter app architecture.
How to simplify the Flutter skeleton app template to more easily access localized strings inside our widgets.
An in-depth overview of the repository pattern in Flutter: what it is, when to use it, and various implementation strategies along with their tradeoffs.
When performing asynchronous work, we need to account for loading and error states in our UI. This article presents simple and reusable approach to handle this across multiple screens.
How to parse large JSON data using compute, Isolate.spawn, and Isolate.exit - a new feature for fast concurrency with worker isolates in Dart 2.15.
How to implement a responsive layout in Flutter by using a split view on large screens and drawer navigation on mobile.
GridView is only suitable for items with a fixed aspect ratio. Here's how to use the flutter_layout_grid package to render responsive layouts with variable item sizes.
And in-depth performance comparison of my new home page, built with Flutter web vs standard web technologies. All benchmarks were run with Google PageSpeed Insights and WebPageTest.org.
Let's build a simple mood tracking app and learn how to work with Firestore, Cloud Functions, and the Firebase Local Emulator.
Learn how to use the Flutter TabBar widget to take the user through a sequence of pages, and disable user interaction on the tabs themselves.
A tutorial about text input validation, showing how to work with TextField, TextEditingController, Form, TextFormField as well as their tradeoffs.
How to create a reusable widget class that helps us when working with asynchronous data from Riverpod providers.
A simple and elegant approach to build a Chat Messaging UI with DecoratedBox, Align, and Padding widgets in Flutter.
An overview of Flutter's built-in widgets for managing state, along with links to the best resources from the official Flutter documentation.
A detailed overview of a production-ready architecture that I've fine-tuned over the last two years. You can use the included starter project as the foundation for your Flutter & Firebase apps.
How to animate a custom task completion ring using AnimationController and AnimatedBuilder in Flutter.
CustomPainter is often the way to go when we need to draw some custom shapes in Flutter. Let's see how to use it in practice.
Migrating Flutter apps to Null Safety can be a challenging process. Here I show how to make this as painless as possible, using a non-trivial app as an example.
Need a Flutter widget that updates every frame? Here's why using Timer is not a good choice, and why Ticker is a much better solution.
Tired of writing JSON parsing code by hand? Here's how to automate this with code generation and the Freezed package.
Mutating state or calling async code inside the build method can cause unwanted widget rebuilds and unintended behaviour. Here are some examples and rules to follow.
How to create a custom animation curve and implement a shake effect that can be applied to any widget in Flutter.
Learn how to use the most common Flutter animation APIs with examples and a free gallery app on GitHub.
A complete tour of Null Safety & non-nullable types, the syntax changes they introduce in Dart 2.12, and how to use them in practice.
How to use animations in Flutter to spice up a fun game written in Dartpad. Learn about implicit animations, AnimatedAlign, duration and curves.
Why and when to use the child widget argument to optimize Flutter performance when working with TweenAnimationBuilder and AnimatedBuilder.
How to use Flutter Hooks or State subclassing to reduce your AnimationController boilerplate code.
Going from RGB to HSL: How to more easily reason about colors as hue, saturation and lightness, and how to use HSL in Flutter.
How to build an interactive page flip widget using Flutter's AnimationController, AnimationBuilder, gesture detectors and custom 3D matrix transforms (part 2).
How to build an interactive page flip widget using Flutter's AnimationController, AnimationBuilder, gesture detectors and custom 3D matrix transforms (part 1).
Many Material widgets such as InkWell, ElevatedButton, and ListTile show a splash effect when selected. Here's how to disable this.
Mixing UI and logic inside Flutter widgets is bad. Here's how to refactor a simple app for better separation of concerns, immutability, and type safety using Freezed & State Notifier.
Two effective techniques for reducing code generation times for Flutter apps that use build_runner.
Should you choose Flutter or React Native for your next app? This article offers an in-depth overview of the two frameworks, along with their pros and cons.
I published a directory of all my open source Flutter apps and projects on GitHub. Here's where to find it.
A guide to implementing multiple independent navigation stacks with BottomNavigationBar in Flutter.
A curated list of useful Dart tips that will improve your coding style as a Flutter developer.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
What implicit downcasts are, why they make your code unsafe, and how to avoid them.
How to create your own Dart packages from existing apps, and other things you need to know.
8 top tips that will save you time in your Flutter web projects.
Best practices for implementing search with RxDart in Flutter, using the GitHub Search REST API as an example.
In-depth tutorial explaning combineLatest and data modeling with movie favourite example Flutter app.
A useful tip to hide your Firebase config from git in your Flutter web projects.
Take-home projects are a task often given to candidates during the interview process. This article shows a hypothetical assignment that I would give to candidates interviewing for a Flutter developer role.
This tutorial shows how to use Flutter custom painters to draw a happy face on screen with Dartpad, starting from scratch. Included: drawing custom shapes with Canvas and Paint, and layout considerations when using CustomPainter.
FocusScopeNode provides a simpler way of move the focus between text fields in your Flutter forms.
How to remove some noise in your Dart code by using underscores for unused function arguments.
How to make your ListViews feel native on iOS by adding top and bottom separators.
CodeWithAndrea.com is a brand new website about high-quality Flutter tutorials. It will contain all my YouTube videos, articles and courses.
Evaluating Flutter from various criteria: Portability, Language Tooling and Features, Documentation, Performance, Testing, Community, Package Ecosystem, Maintainer Commitment and many more.
Dart extensions unlock a few interesting use cases in Flutter apps. This tutorial shows how to enable them, what they are, when to use them, and when not to.
Let's see how to write integration tests with Flutter Driver, how they differ from widget tests, and how to run them with Codemagic.
In-depth overview of Slivers and how to use them (part 2). Includes a demo app showing how to use SliverList, SliverGrid, SliverToBoxAdapter, SliverFillRemaining.
In-depth overview of Slivers and how to use them (part 1). Includes a demo app showing how to use SliverAppBar and SliverPersistentHeader.
Overview of the 'Dart as UI' features introduced in Dart 2.3. Uses a fitness tracker custom UI as an example.
Overview and practical use cases of type inference, final & const, named & positional parameters, @required & default values.
Let's see how use service classes to encapsulate 3rd party libraries and APIs, and decouple them from the rest of the application. We will use authentication as a concrete example of this.
This article shows how to use scoped access with Provider when using service classes in our Flutter apps.
In this article we implement a simple authentication flow in Flutter, in less than 100 lines of code.
This article introduces a new architectural pattern that I often use in my Flutter Apps. It is inspired by BLoCs and RxVMS.
Detailed side-by-side comparison of language features between Dart 2.1 and Swift 4.2.
Clarifications and apologies following my previous article.
Controversial article with some history and a detailed comparison between Flutter and native iOS development. Includes an example showing how to build a contact list in Flutter and iOS.
Tutorial showing how to switch between Material and Cupertino widgets, and increase code reuse by building a platform-aware abstract base class with concrete sub-classes. Includes an overview of dialogs and their platform-specific differences.
Flutter is awesome! Big thanks to the Flutter team and all the people in the wider community that keep pushing this project to new heights and sharing new learning material.
Let's learn about color gradients, composition, writing reusable UI code, and CupertinoSegmentedControl.
Let's see how to load a CSV file into strongly-typed model classes, that can be used to calculate CO2 emissions for flights between two airports.
Overview of regular expressions (regex). How to implement a Regex validator in Dart and integrate it with Flutter's TextField widget, so that we can validate user input.
Let's see how to use some custom code to add animated overlays and reveal action options from a FAB.
How to dock a floating action button in the middle of a BottomAppBar.
Deep dive into widget tests. Introduces WidgetTester, Finder, matcher objects, and shows how to write tests for a login screen. Includes test mocks, mockito, acceptance criteria.
How to extract business logic from your apps into testable classes, and write unit tests in Flutter. Uses a login demo example to write email & password validation tests.
How to build layouts with scrollable pages, lists, grids, and other convenience widgets. Includes slivers and their usage to create a hero image effect.
List of the most useful IntelliJ/Android Studio shortcuts for better productivity.
How to build basic layouts in Flutter. Also included: MainAxisAlignment, MainAxisSize, CrossAxisAlignment, Baseline, AlignmentDirectional, LayoutBuilder, SizedBox.
Let's see how to use platform channels by building an image picker on iOS, and using it from the Dart code.
This article shows how to write testable code in Flutter, and take your widget tests to the next level.
In-depth performance comparison for a stopwatch app built in native iOS vs Flutter.