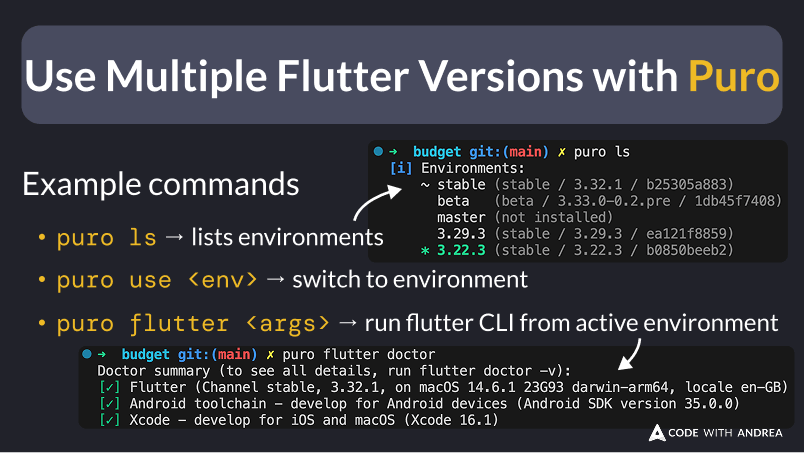
Use Multiple Flutter Versions with Puro
1 min read
Puro is a powerful tool that lets you install multiple versions of Flutter and easily switch between them in your projects.
Puro is a powerful tool that lets you install multiple versions of Flutter and easily switch between them in your projects.
Dart 3.8 introduces a new formatting option to preserve trailing commas, overriding the new formatting style introduced in Dart 3.7.
Null-aware elements allow you to add nullable elements to a collection with a single character (?).
To enable this, switch to Flutter 3.32 and run your app with --web-experimental-hot-reload
If your app uses GoRouter with shell routes, tracking screen views reliably requires some workarounds. Here's how to make it work.
Material 3 supports 5 types of buttons: elevated, filled, filled tonal, outlined, and text. Here's how to use them in Flutter.
By overriding ThemeData.platform inside MaterialApp, you can quickly test all your adaptive widgets and any conditional code that checks the platform.
A simple alert dialog that supports adaptive mode, default and cancel actions, destructive style, and dismissible mode.
Use this script to update the Gradle, Java, NDK version and other settings in your Android project.
A battle tested solution for loading Firebase Remote Config in Flutter, avoiding common pitfalls.
debugRepaintRainbowEnabled helps you discover widgets/areas that unexpectedly repaint in your app. Here's how to use it.
debugRepaintRainbowEnabled helps you discover widgets/areas that unexpectedly repaint in your app. Here's how to use it.
When working with forms in Flutter, numeric inputs need special attention. To improve the user experience, set the appropriate keyboardType and inputFormatters.
The TextSpan class lets you set a custom mouse cursor style, along with a tap gesture recognizer for opening your URL links on Flutter web.
A/B tests help you make data-driven decisions and increase conversions in your app. Here's how they work.
Static release toggles let you release unfinished code without activating it in production. Here's how to use --dart-define to manage them.
When reading variables from .env files, you can use int.fromEnvironment and bool.fromEnvironment to read integers and booleans.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Instead of taking screenshots manually for each device & language, you can automate it with Maestro! Here's how.
Here's a handy command to override the Android status bar UI before taking screenshots for your Play Store listings.
Here's a handy command to override the iOS status bar UI before taking screenshots.
Apps created with Flutter 3.29 use the new Gradle Kotlin DSL on Android. Some CLI tools don't support the new syntax, yet.
Pub.dev has a new chart that lets you see package download counts by version (major, minor, patch). Here's where to find it.
Pub.dev has a new chart that lets you see package download counts by version (major, minor, patch). Here's where to find it.
By registering a ValueNotifier listener, you can perform side effects such as updating controllers, showing dialogs, and performing navigation.
Dart 3.7 introduces a new formatter that automatically adds or removes trailing commas, based on the max line length.
Since Dart 3.7, the _ character is a wildcard variable and you can use it more than once in your code, without causing name collisions.
The CallbackShortcuts widget makes it easy to add keyboard shortcuts to your Flutter app. Here's how to use it.
Here's how to create a responsive split-view widget that works on mobile, desktop and web.
If you're using GitHub Actions for your CI/CD needs, you can setup a self-hosted runner to cut your build times and save money.
If you add a debugFillProperties() method to your custom widget classes, all your custom widget properties will show in the DevTools.
If you enable code obfuscation in your Flutter release builds, the stack traces will be unreadable in the Sentry crash reports. Here's how to fix it.
If your Codemagic builds are failing, you can enable SSH access and login to the build runner to diagnose the issue.
With VSCode, you can easily move your Dart classes and functions to a different file (this is very useful when refactoring).
Here's how to overlay multiple widgets inside a Stack and constrain their size and position with Positioned and FractionallySizedBox.
If you need to select between a small list of values but have limited vertical space, you can use a ListWheelScrollView.
To support the latest wide gamut color spaces, Flutter 3.27 has deprecated some properties and methods in the Color class.
If you want to render fixed width (monospaced) digits, set FontFeature.tabularFigures() inside your TextStyle.
Since Dart 3.6 (Flutter 3.27), you can use _ as a digits separator. This works with integers and floats, as well as custom formats (hex, scientific).
Since Flutter 3.27, you can pass a spacing argument to your Row and Column widgets (rather than using SizedBox).
You can use the Banner widget to place a small diagonal banner over a child widget. For more custom styling, build your own using a custom painter.
You can use Cursor edit mode to sweep through your code and make changes. Works best with imperative code.
A few suggestions for solving the "version solving failed" error when updating your Flutter dependencies.
Starting November 12, 2024, apps that don’t include a Privacy Manifest can’t be submitted for review in App Store Connect.
If your app does not use Non-Exempt Encryption, set ITSAppUsesNonExemptEncryption to NO in your Info.plist file in Xcode.
Upgrade all your file icons with the Material Icons Theme. File nesting is properly supported, too, and the icons will be correctly left-aligned.
A simple script to build and upload your iOS app to App Store Connect. You can run this locally, no CI/CD needed!
Some guidelines to help you decide which API keys belong on the client, and which belong to the server.
How to enable dark mode and see downloads count for your favourite packages on pub.dev.
With Error.throwWithStackTrace, you can throw custom exceptions while keeping the original stack trace intact.
If your app sales are less than $1M/year, you can apply to the Small Business Program and slash your fees to 15%!
Since Riverpod 2.6.0, all generated providers can be declared with a Ref argument. Here's how to migrate to the new syntax.
If you want to upgrade all dependencies to the latest non-major version, ignoring the pubspec.lock file, use flutter pub upgrade.
An overview of two different strategies for initializing Firebase inside a Flutter app with multiple flavors.
If you have a Flutter project that no longer builds on a specific platform, you can delete the whole folder and generate it again.
If you ever needed a force update prompt that is controlled remotely, you can use the force_update_helper package.
Your Flutter app should show the licenses for packages in use. This is often a legal requirement, as many open-source licenses require attribution.
How to enable dark and tinted icons on iOS 18 using the flutter_launcher_icons package.
If your Flutter app uses multiple flavors, you can use the FlutterFire CLI to generate the config files for each flavor.
Here's how to remotely control the behaviour of your app by fetching some JSON from a GitHub gist.
If you're not too picky about how you write your commit messages, this can be a neat little time saver!
If you're using multiple code generators that depend on each other, you can enforce the code generation order in your build.yaml file.
If you want to return a stream that depends on some asynchronous code, you can use async* and yield*
To avoid prompting users too early, track your desired event and only ask for a review after it is triggered N times.
How to download multiple releases from xcodereleases.com, and switch between them with the xcode-select CLI.
By implementing a NavigatorObserver, you can track page views or add navigation breadcrumbs to your error logs.
The Android debug logs can get quite noisy. To work around it, open the DevTools logging page, which will only show the Flutter logs.
Rather than calling Navigator.of(context), you can define all the push/pop methods inside a BuildContext extension and make your navigation code less verbose.
To create a stylish Text, use the ShaderMask widget with a shaderCallback that returns a LinearGradient with begin and end points, along with a list of colors.
The easiest way to add a badge to an IconButton is to use the Badge widget. Use this to show a numeric value or a custom label next to an icon.
Thanks to RawGestureDetector and SerialTapGestureRecognizer, you can implement a custom TripleTapDetector widget.
How to use the Flutter VSCode sidebar to access the DevTools and other useful functionality.
Since Flutter 3.24, a new Rebuild Stats feature is available on the DevTools performance page. Use it to spot widgets that rebuild too often.
Since Flutter 3.24, a new CarouselView widget is available. You can set the children's size with itemExtent and shrinkExtent, and use it with any widgets as children.
As of Flutter 3.24, a new "pub unpack" command is available. You can use it to download a package locally and easily explore its source code.
OverflowBar makes it easy to layout your widgets in a row unless they overflow the available horizontal space, in which case they're arranged as a column.
When you call a method that returns a Future, you have to choose between using await, unawaited, and ignore. Here's an explanation.
The humble log function has many arguments that can be used to customize the appearance of your logs. Here's how to use them.
When tracking analytics events in your code, consider using `unawaited` from `dart:async` (analytics calls should be fire & forget).
--dart-define-from-file supports both .env and json files. Here's how to use each variant.
By doing some string manipulation on the current stack trace, you can extract the current method name (useful for logging).
The hugeicons package was recently released, featuring a collection of over 4,000 stroke Flutter icons.
You can use CancelToken to stop unnecessary data fetches when users navigate away or to implement "cancel" buttons in your Flutter apps.
You can use BoxHeightStyle to change the selection height style of a TextField (useful for multi-language apps).
The widget build method can potentially be called in every frame and should not have any side effects.
If you need to show a semi-transparent image, use the opacity argument with AlwaysStoppedAnimation rather than adding a parent Opacity widget.
Forgetting to dispose controllers in your State class can lead to memory leaks, and the "dispose-fields" rule from DCM can warn you about it.
With this VSCode extension, you can remove all the unused assets, files, and dependencies in your Flutter project and decrease your app bundle size.
If you try to access a web-specific API on native platforms, your app will crash. Here's how to use conditional imports to solve this problem.
To detect the current platform, check for kIsWeb beforehand and use it together with defaultTargetPlatform, which doesn't import dart:io.
With the Universal Platform package, you can perform platform detection with a unified syntax on all platforms, including web, but there's a catch.
A collection of useful aliases to speed up your Flutter app development workflow.
How to use the Flutter CLI to save screenshots from connected iOS and Android emulators
Here's how to use typedef to define type aliases for your function and non-function types in Dart.
By adding type annotations to your collections, the Dart analyzer will warn you if you add values of the wrong type.
Const is for hardcoded, compile-time constants. Final is for read-only variables that are set just once. Var is for variables that are set more than once.
How to use the web app bootstrap process (new in Flutter 3.22) and add a CSS progress indicator before the Flutter app is ready to take over.
Here's how to use the Vector Graphics Compiler to precompile SVGs at build time for better rendering performance.
How to use the JsonCodable macro to augment your classes with fromJson and toJson methods
If you need to return an empty widget, SizedBox.shrink() is more performant than an empty Container.
By default, asserts are only enabled in Debug mode. To enable them in Release mode, run with the --enable-asserts flag.
How to send HTTP requests and view the response directly in Visual Studio Code.
If you use build_runner and your generated Dart files are added to Git, you can hide them by default in your PRs and diffs. Here's how.
Some helper widgets for creating Flutter layouts that grow horizontally up to a given width, then remain fixed at that width.
Ever wanted to filter tests so you only run the ones you need to? This can be easily done using test tags.
You can run a widget test multiple times with the variant argument. Very useful for golden image tests for different screen sizes.
Create small, reusable widgets that are easier to reason about, and banish the massive build method from existence!
In Flutter you can use DecoratedBox to set a lot of decoration/styling options to your widgets. Here's how.
The faker package lets you generate addresses, names, food, dates, sports... you name it! Here's how to use it.
A useful tip to write more performant code when using SizedBox as a gap between widgets inside a Row or Column layout.
If you have many AsyncNotifier subclasses, using try/catch can be tedious. With AsyncValue.guard you get the same result with less boilerplate.
How to style an ElevatedButton in Flutter, including reusing the same style across all buttons with ThemeData.
How to add a GitHub Actions workflow to automatically run tests when you push your code.
How to easily add fixed spacing inside Flex widgets such as Columns and Rows or scrolling views.
Since Flutter 3.7, BuildContext has a mounted property that we can check after an asynchronous gap. Here's how to use it.
An overview of what Dart Fix can do for you, along with some useful VSCode productivity tips and settings for Flutter app development.
Flutter apps built for macOS need a client network entitlement in order to make network requests. Here's how to configure it.
DartPad makes it easy to share your Dart & Flutter samples using GitHub gists, and you can even embed them on your website. Here's how.
When a test waits for a stream value that is never emitted, it will timeout after 30 seconds (by default). Here's how to make it fail fast with a custom timeout.
Since Dart 2.17, you can initialize parameters of the super class with a new shorthand syntax. Here's how.
Since Dart 2.17, we can add members and additional methods when declaring an enum. Here's how.
When writing tests for functions that throw, we should not invoke them directly, but rather pass them as arguments using a tear-off.
Ever needed to update a value if a given key already exists, or set it if it doesn't? Here's how to use the Map.update() method to solve this.
How to use the precompiled Firestore iOS SDKs to speed-up Xcode builds on your Flutter apps.
Many Material widgets such as InkWell, ElevatedButton, and ListTile show a splash effect when selected. Here's how to disable this.
Two effective techniques for reducing code generation times for Flutter apps that use build_runner.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
Easy ways to improve your Dart & Flutter code. Published weekly.
A useful tip to hide your Firebase config from git in your Flutter web projects.
FocusScopeNode provides a simpler way of move the focus between text fields in your Flutter forms.
How to remove some noise in your Dart code by using underscores for unused function arguments.
How to make your ListViews feel native on iOS by adding top and bottom separators.