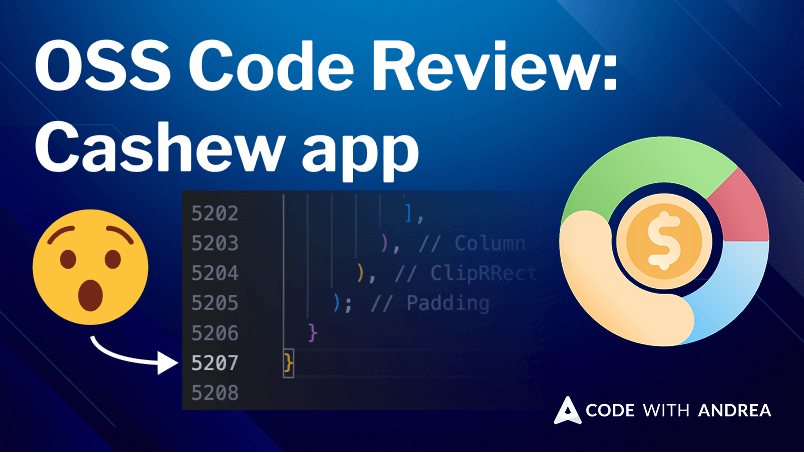
Code Review of Cashew App: Lessons from a Flutter App with 100k+ Downloads
10 min read
In-depth code review of Cashew, a budget and expense tracking app with 4.9 star ratings, 100,000+ downloads, and just as many lines of code.
In-depth code review of Cashew, a budget and expense tracking app with 4.9 star ratings, 100,000+ downloads, and just as many lines of code.
A video showing how to create a custom app icon from scratch in Figma, even if you're new to design.
An overview of Firebase as a BaaS platform, including available features, supported platforms, pricing, portability, documentation, and developer experience.
And in-depth performance comparison of my new home page, built with Flutter web vs standard web technologies. All benchmarks were run with Google PageSpeed Insights and WebPageTest.org.
How to implement Apple Sign In with Flutter & Firebase Authentication (from scratch), and give your iOS users a convenient way of signing into your app.
An overview of Flutter's built-in widgets for managing state, along with links to the best resources from the official Flutter documentation.
A detailed overview of a production-ready architecture that I've fine-tuned over the last two years. You can use the included starter project as the foundation for your Flutter & Firebase apps.
Learn how to use the most common Flutter animation APIs with examples and a free gallery app on GitHub.
Tips for learning how to build your own Flutter apps, overcome Tutorial Hell, and figure out what to do when you get stuck.
A complete tour of Null Safety & non-nullable types, the syntax changes they introduce in Dart 2.12, and how to use them in practice.
How to use animations in Flutter to spice up a fun game written in Dartpad. Learn about implicit animations, AnimatedAlign, duration and curves.
How to use Flutter Hooks or State subclassing to reduce your AnimationController boilerplate code.
How to build an interactive page flip widget using Flutter's AnimationController, AnimationBuilder, gesture detectors and custom 3D matrix transforms (part 2).
How to build an interactive page flip widget using Flutter's AnimationController, AnimationBuilder, gesture detectors and custom 3D matrix transforms (part 1).
Mixing UI and logic inside Flutter widgets is bad. Here's how to refactor a simple app for better separation of concerns, immutability, and type safety using Freezed & State Notifier.
I published a directory of all my open source Flutter apps and projects on GitHub. Here's where to find it.
A curated list of useful Dart tips that will improve your coding style as a Flutter developer.
How to improve your coding skills and become a better software engineer.
How to create your own Dart packages from existing apps, and other things you need to know.
8 top tips that will save you time in your Flutter web projects.
Best practices for implementing search with RxDart in Flutter, using the GitHub Search REST API as an example.
In-depth tutorial explaning combineLatest and data modeling with movie favourite example Flutter app.
Take-home projects are a task often given to candidates during the interview process. This article shows a hypothetical assignment that I would give to candidates interviewing for a Flutter developer role.
This tutorial shows how to use Flutter custom painters to draw a happy face on screen with Dartpad, starting from scratch. Included: drawing custom shapes with Canvas and Paint, and layout considerations when using CustomPainter.
Evaluating Flutter from various criteria: Portability, Language Tooling and Features, Documentation, Performance, Testing, Community, Package Ecosystem, Maintainer Commitment and many more.
Dart extensions unlock a few interesting use cases in Flutter apps. This tutorial shows how to enable them, what they are, when to use them, and when not to.
In-depth overview of Slivers and how to use them (part 2). Includes a demo app showing how to use SliverList, SliverGrid, SliverToBoxAdapter, SliverFillRemaining.
In-depth overview of Slivers and how to use them (part 1). Includes a demo app showing how to use SliverAppBar and SliverPersistentHeader.
Overview of the 'Dart as UI' features introduced in Dart 2.3. Uses a fitness tracker custom UI as an example.
Overview and practical use cases of type inference, final & const, named & positional parameters, @required & default values.
Tutorial showing how to switch between Material and Cupertino widgets, and increase code reuse by building a platform-aware abstract base class with concrete sub-classes. Includes an overview of dialogs and their platform-specific differences.
Overview of regular expressions (regex). How to implement a Regex validator in Dart and integrate it with Flutter's TextField widget, so that we can validate user input.
Deep dive into widget tests. Introduces WidgetTester, Finder, matcher objects, and shows how to write tests for a login screen. Includes test mocks, mockito, acceptance criteria.
How to extract business logic from your apps into testable classes, and write unit tests in Flutter. Uses a login demo example to write email & password validation tests.
How to build layouts with scrollable pages, lists, grids, and other convenience widgets. Includes slivers and their usage to create a hero image effect.
How to build basic layouts in Flutter. Also included: MainAxisAlignment, MainAxisSize, CrossAxisAlignment, Baseline, AlignmentDirectional, LayoutBuilder, SizedBox.