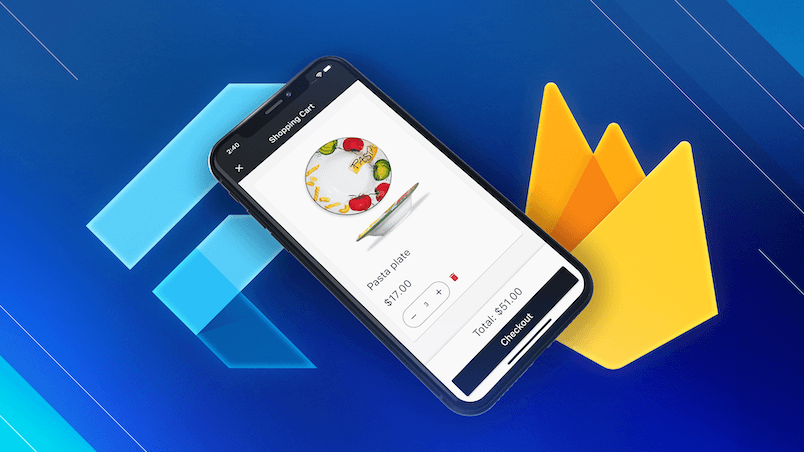
Full Course
Flutter & Firebase Masterclass
Learn about Firebase Auth, Cloud Firestore, Cloud Functions, Stripe payments, and much more by building a full-stack eCommerce app with Flutter & Firebase.
Andrea’s Flutter content is amazing - it’s one of the few resources I consistently reference for advanced real-world solutions.
He clearly puts a huge amount of care and thought into every tutorial. Highly recommended!
Learn More
Course Overview
If you need a backend for your Flutter app, Firebase is the most obvious choice.
Firebase makes it easy to authenticate users, write data to a remote database, and run server-side code securely. And you get scalability and great performance out of the box, without breaking the bank.
But once you start building large-scale apps, things get complicated and you may ask yourself:
- Do I have the right architecture in place?
- Am I structuring my data correctly?
- How can I run complex queries efficiently?
- Are my security rules correct?
- How should I handle errors?
- Will I get a massive Firebase bill and go bankrupt?
In other words - how do you know if you’re doing it right?
Sure, you could read all the docs and watch a ton of tutorials on YouTube - but those often take shortcuts or use examples that are too basic and don't give you the full picture.
As a result, you’re left to learn the hard stuff by trial and error, and that takes ages!
But there's a faster way.
And I can speed up the process for you, by packaging up all my Firebase knowledge and experience into this course.
It's time to
Learn Firebase
This in-depth course will teach you how to create real-world apps with Flutter & Firebase.
Start with the Basics, Then Dive Deep
We'll kick off by building a simple full-stack app that covers Firebase Auth, Cloud Firestore, basic security rules, and the latest Firebase UI packages.
Then, we’ll go deep and build a complex eCommerce application, complete with backend integration using Cloud Functions and 3rd party integrations like Stripe and Algolia.
Along the way, we’ll cover key topics such as:
- Firebase setup with the FlutterFire CLI
- Authentication flows and role-based authorization
- Data modeling, security rules, and CRUD operations with Cloud Firestore
- Queries, indexes, pagination, caching, and offline support
- Image uploads with Firebase Storage
- Firebase Local Emulator and Cloud Functions with TypeScript
- Firebase cost analysis and optimization
- Firebase Extensions and 3rd party integrations (Stripe and Algolia)
- How to securely store client-side and server-side API keys
- Deployment via Firebase Hosting
Course Format
The course contains a variety of code-along videos, along with diagrams and illustrations to help you understand the concepts, as well as full source code, written notes, and additional resources that you can reference later.
Here’s what my past students had to say: 👇
Loved the course and got a lot out of it.
Especially appreciated how Andrea doesn't just show you the "right way" to do things from the start. He shows common errors and pitfalls and how to get around them, and eventually end up at the "right way" with a better understanding of how to actually get there in your own projects.
The course also has a healthy balance of code-alongs for relevant portions and provides you with updated project files throughout, so you don't have to spend hours manually going through every single line of the project.
I finally finished Andrea's two main courses. It is hard-core and very intensive; unlike the other introductory courses, Andrea's courses are very clear and hit the objective directly.
Most importantly, the quality of the code is very high and is also based on the most updated SDK versions. Just looking through the code makes me feel very pleasant.
It's only my first time going through the code; I'll do it more times and try to finish the app, not following the course closely.
Course Curriculum
What's Inside
Introduction
Firebase Pros and Cons
An overview of the Firebase Pros and Cons for Flutter app development:
- List of features and supported platforms
- Dart support (client vs server-side)
- Pricing
- Portability & vendor lock-in
- Developer experience
Module 1
Firebase Basics
Create a simple full-stack app with Flutter and Firebase:
- Installing the Firebase and FlutterFire CLI tools
- Adding Firebase to a Flutter app
- Simple authentication flow with Firebase UI
- Listening to auth state changes with GoRouter and Riverpod
- Getting started with Cloud Firestore
- CRUD operations and reading realtime data
- Firebase UI for Firestore: FirestoreListView, FirestoreQueryBuilder, Pagination
- Caching and offline mode
- Security rules and finishing touches
Module 2
Full-Stack eCommerce App Overview
A full overview of the eCommerce starter project:
- Feature-first app architecture with Riverpod
- Using repositories as Firebase API wrappers
- Choosing real vs "fake" repositories with provider overrides
- Configurable app bootstrap code
- Project structure: features and layers
- Modular architecture and Firebase UI tradeoffs
Module 3
Firebase Authentication
Adding user authentication flows to the app:
- Introduction to Firebase Authentication
- Adding Firebase to the eCommerce app (using FlutterFire CLI)
- Abstracting the FirebaseAuth APIs behind a repository
- Sign in with email and password
- Auth state changes and the User class
- Dealing with FirebaseAuthExceptions
- How to implement email verification
- Automated testing with FirebaseAuth
Module 4
Cloud Firestore and Firebase Storage
Multi-page image upload workflows with Firebase Storage and Cloud Firestore:
- Overview of the image upload UI screens
- Uploading images with Firebase Storage
- Storing the download URL with Cloud Firestore
- Adding, editing, and deleting products with Cloud Firestore
- Alternative image upload workflows: file picker / image picker
- User input and validation with complex forms
- Firebase error handling
- CORS setup for Firebase Storage images on Flutter web
Module 5
Firebase Local Emulator and Cloud Functions
Adding custom server-side code with Cloud Functions:
- Adding a local Firebase project with the Firebase CLI
- Working with the Firebase Local Emulator (including importing and exporting data)
- Introduction to Cloud Functions and TypeScript
- TypeScript ESLint configuration
- Writing and testing Cloud Functions locally with hot reload
- Cloud Functions triggers
- Preventing infinite loops and recursive cloud function calls
- Writing and HTTP callable functions and invoking them from Flutter
- Deploying Cloud Functions and monitoring them with the Logs Explorer
- Setting up Google Cloud budget alerts
Module 6
Role-Based Authorization with Custom Claims
Implementing role-based authorization with custom claims:
- What are custom claims and what problems they solve
- High-level overview: server-side logic, client-side logic, and security rules
- Writing a Firebase Auth Trigger to set custom claims on the server
- Enforcing security rules based on the Firebase ID token
- Unlocking admin-only features in the Flutter app with custom claims
- How to combine custom claims and realtime listeners to improve the user experience
- Deploying and testing the app with the real Firebase backend
- Alternative use cases and variants for role-based authorization
Module 7
Diving Deeper with Cloud Firestore
Diving deeper with data modeling, realtime updates, and cost optimization:
- NoSQL data modeling
- Setup complex realtime data flows from Firestore to Flutter
- Combining values from different Firestore documents using providers
- Firebase cost measurement and optimization
Module 8
Stripe Integration
How to build a seamless checkout experience using Stripe:
- Introduction to Stripe and other payment processors
- An overview of the Flutter Stripe SDK and example app
- How to install and configure the Firebase Stripe extension
- Webhooks: what they are and how to configure them
- How to use Cloud Function triggers to sync data between Firestore and Stripe
- Client payment flows with native payment sheets on iOS & Android
- Flutter web payments with Stripe checkout and redirects
- Server-side order fulfillment
- How to handle Stripe API keys securely on the client and the server
Module 9
Full-Text Search with Algolia
How to add full-text search with Algolia, Flutter, and Firebase to our eCommerce app:
- Overview of the Firebase Extensions for Full-Text Search
- Installing the Algolia Extension for Firebase
- Adding full-text search to the Flutter app with a modular architecture
- Using caching and debouncing to make fewer API calls
- How to add realtime updates to the search implementation
Module 10
Completing the eCommerce app
Completing the eCommerce app and deploying it as a Flutter web demo using Firebase Hosting:
- Reading and writing product reviews with Cloud Firestore
- Introduction to Cloud Firestore aggregate queries (COUNT, SUM, AVG)
- Calculating the average product rating with a Cloud Function trigger
- Deploying the Flutter web app with Firebase Hosting (CORS setup included)
We’ll cover the topics above in great detail, and you won’t be left wondering if your code is secure or if you've structured your database properly.
And when it comes to code quality, we’ll make sure to write clean, maintainable code that’s easy to extend and scale.
The bottom line?
By the end of this course, you'll have the knowledge and skills needed to build full-stack apps with Flutter and Firebase. So don't miss out on the opportunity to fast-track your app development journey. 🔥
I just started this class a couple of days ago, Andrea, and I am already very impressed. Although I usually go to Udemy and have found some good instructors there, this class is truly next-level.
Your intelligence is evident, but what really impresses me is your ability to simplify complex concepts. Your attention to detail, including your thoughtful approach to code architecture, sets you apart. Well done!
Very good course and everything works as explained by Andrea.
I like the detailed explanation of why he is suggesting a particular course of action.
Excellent resource for Flutter developers.
Get the Flutter & Firebase Masterclass
Updated May 2024. Works with the latest Flutter and Firebase versions.
Join 1,000+ developers who have already decided to advance their skills.
Complete Package
- All 10 modules, totaling 12 hours of video
- Lifetime Access
- All Bonus Articles
- Full Source Code
- Premium Support on Discord
- English Subtitles
- Completion Certificate
- 30 Day Money Back Guarantee
The Essentials
- First 5 modules, totaling 7 hours of video
- Lifetime Access
- Some Bonus Articles
- Full Source Code
- Premium Support on Discord
- English Subtitles
- 30 Day Money Back Guarantee
Checkout powered by LemonSqueezy.
Team packages are also available. For more details, see this page.
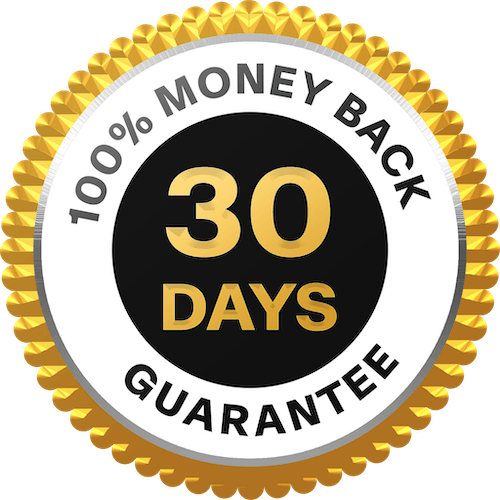
I spent over 600 hours making this a high-quality course and I'm confident you'll get a lot of value out of it.
But the only way to find out if this course is right for you is on the inside, not on the outside. So you get on the inside and see if it’s true and valuable to you. Then, if it is, that’s when you decide to keep it.
But if you're not happy with it, for any reason, you can reach out within 30 days of purchase to get a full refund. All you have to do is go to courses@codewithandrea.com and tell me “gimme my money back” and you got it, and in short order. My response time is usually 24 hours.
Andrea has some of the highest quality online courses I have seen. He does not gloss over the issues of optimization or best practices that so many other courses do.
You can adapt what you learn from his courses, right into real-world applications!
So far, the course has delivered its promise to teach from a practical and professional perspective for intermediate and advanced users.
Love the tips and the side notes on the lessons.
The content is simply exemplary. If you are interested in creating cross-device apps, then Flutter is a great choice and Andrea's lessons will get you up to speed.
If you care about good architecture, Andrea will help you out with a sensible approach that will make your code readable and maintainable.
I'm starting to realize that I was doing things the wrong way from the beginning.
I'm excited to see that I'm finally learning the best practices for working on a Flutter app, and I'm sure I will become very confident about building the complex app that I need.
Intended Audience
Who is this course for?
This is an intermediate to advanced-level course about Flutter & Firebase, and you need to be already familiar with Dart, Flutter, GoRouter, and Riverpod to make the most of it.
We’ll start by building a simple full-stack app so you'll get comfortable with the basics even if you never used Firebase before.
Then, I’ll give you a detailed walkthrough of the complete eCommerce app that was covered in the Flutter Foundations course. And we’ll turn it into a full-stack app, using the Firebase Dart packages on the frontend, and Cloud Functions with TypeScript on the backend.
When adding new features, I'll include most of the UI code beforehand so we can focus on what matters most: learning Firebase.
While this is not strictly required, I recommend you take the Flutter Foundations course before proceeding to this one.
FAQ
Frequently Asked Questions
How much does the course cost?
The Flutter & Firebase Masterclass costs $149 (unless a promotion is ongoing).
On the checkout page, you'll have the option to add the Flutter Foundations course for an additional $119 ($30 off).
Will you offer discounts in the future?
From time to time (e.g. Black Friday) I offer discounts, however these are very infrequent.
If you want to get value from the course, your best option is to buy today. If you're not satisfied, you can get a refund within 30 days of purchase.
Should I take the Flutter Foundations course before this one?
While this is not strictly required, I recommend taking the Flutter Foundations course before proceeding to this one.
If you wish, you can buy both courses together on the checkout page.
Can I buy all three courses as a single bundle?
This is the second course in a series of three:
- Flutter Foundations Course
- Flutter & Firebase Masterclass
- Flutter in Production
Once the last course is published, you'll be able to buy all three as a single bundle.
But for now, you can buy the first two courses together on the checkout page.
How long is the Flutter & Firebase course?
This course is 12 hours long.
Is the course up to date?
Yes. The course was last updated in May 2024 and is compatible with the latest versions of Flutter and Firebase.
I'll continue to keep it up to date in the future.
Will this course cover all the Firebase products?
Firebase is a big platform that includes many different services to build, release, and monitor your apps. And it would be hard to cover them all in a single course.
So here’s a list of topics that are not planned for this course:
- Firebase Remote Config
- Firebase Dynamic Links
- Firebase Cloud Messaging
- OAuth providers (Google, Apple, Facebook etc.) and advanced authentication features
I will, however, cover some of these topics in future articles or courses.
Will you cover any backends other than Firebase?
This is not currently planned, though I may create additional courses for other backends in the future.
We will design the Flutter codebase with good separation of concerns, making it easier to adopt a different backend if desired.
Will this course cover server-side Dart?
No. We will write Cloud Functions in TypeScript.
If and when official Dart support is added to Firebase Cloud Functions, I'll update the course to reflect that.
What about payment providers other than Stripe?
Stripe and RevenueCat are the only payment processors with good support for Flutter on iOS, Android, and web.
Since we'll build an eCommerce app to sell physical products, Stripe is the ideal choice.
I may cover other payments providers as part of separate courses in the future.
What if I get stuck? Can I ask questions?
Yes. You can ask questions on the course Q/A in the Discord server. I answer most questions within 24 hours.
Will I get a completion certificate?
Sure do! Once you complete the course this will be issued to you automatically.
I'm a student / I can't afford the course / I live outside the US/EU / do you support Purchasing Power Parity (PPP)?
Unfortunately PPP is not supported by my course platform. If you'd like to request a discount, please fill this form.
I bought the essentials package. Can I upgrade to the complete package?
Sure - email me at courses@codewithandrea.com and I'll send you a coupon that you can use to upgrade to the full package.
What if I don't like the course?
The course comes with a 30 day money back guarantee. If you're not happy with the course, for any reason, you can reach out and I'll issue a full refund.
All you have to do is go to courses@codewithandrea.com and tell me “gimme my money back” and you got it, and in short order. My response time is usually 24 hours.
Do you offer team packages?
Sure thing! I offer team packages at discounted rates.
Email me at courses@codewithandrea.com for all the details.
I have another question!
Sure - email me at courses@codewithandrea.com and I'll reply within 48 hours.
instructor
Hello, I’m Andrea
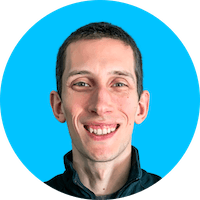
My name is Andrea, I'm a Flutter GDE and I've been writing code professionally for over 15 years. I've been a mobile app developer since 2012, working for startups and big companies.
With my Flutter tutorials and courses, I've helped thousands of students become better developers.
By taking this course, you'll advance your skills and learn how to build full-stack apps with Flutter and Firebase.